“Master Solidity: Ultimate Guide to Smart Contract Development”
“Discover what Solidity is and how to use it effectively. This comprehensive guide covers everything from basics to advanced smart contract development for blockchain platforms.”
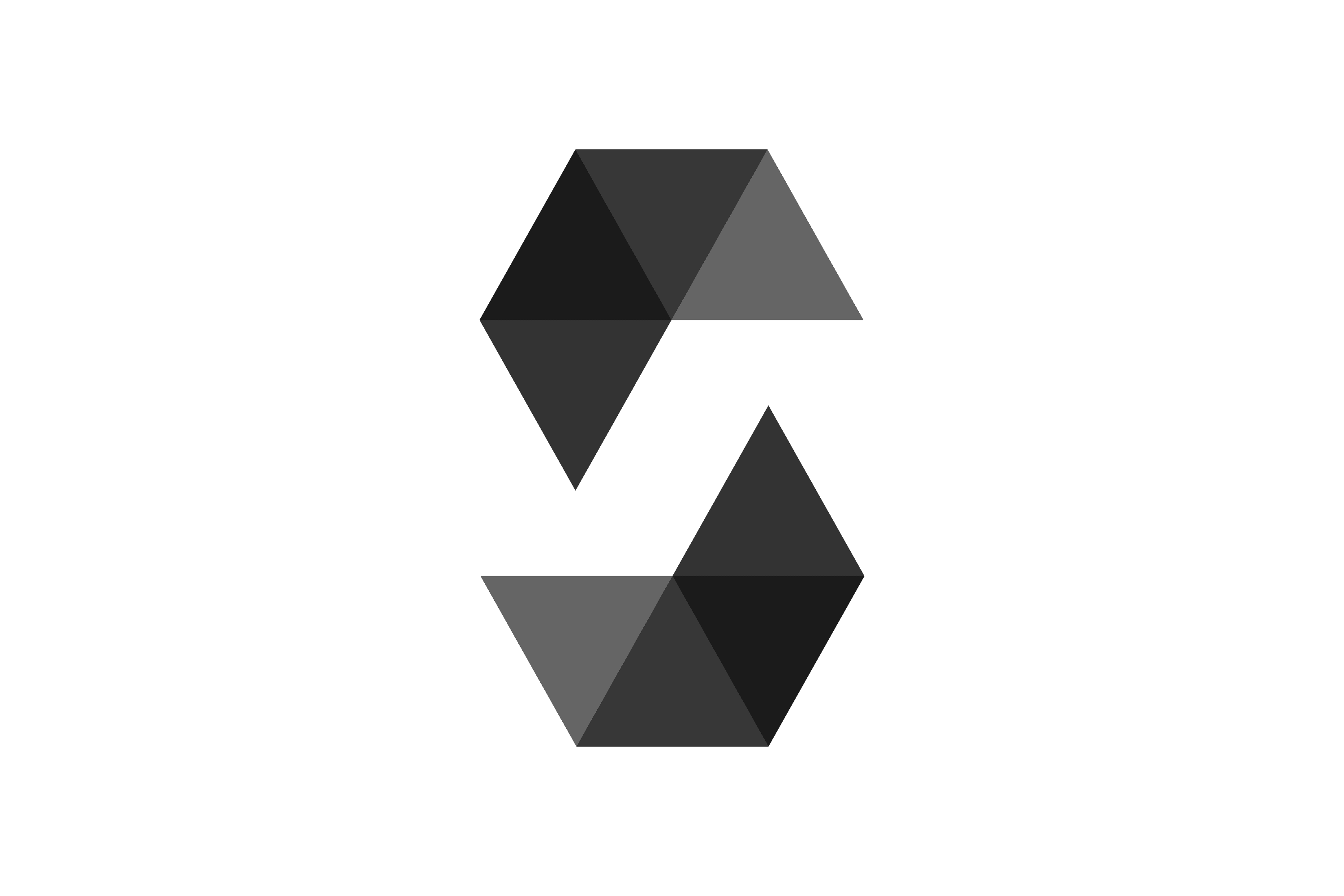
image credits “logo.wine“
Table of Contents
- Introduction to Solidity Programming Language
- What is Solidity Programming Language?
- Why Learn Solidity?
- Key Features of Solidity Programming Language
- Object-Oriented Programming
- Smart Contract Language
- Compatibility with Ethereum Virtual Machine (EVM)
- Setting Up the Solidity Development Environment
- Installing Prerequisites
- Using Remix IDE
- Setting Up Local Development Tools
- Basics of Solidity Programming Language
- Syntax Overview
- Variables and Data Types
- Functions and Modifiers
- Creating Your First Smart Contract
- Step-by-Step Instructions
- Deploying a Contract on Testnet
- Interacting with Deployed Contracts
- Advanced Concepts in Solidity Programming Language
- Events and Logging
- Inheritance in Contracts
- Libraries in Solidity
- Best Practices for Solidity Development
- Writing Secure Contracts
- Gas Optimization Tips
- Code Readability and Documentation
- Tools for Solidity Developers
- Popular Frameworks (Truffle, Hardhat)
- Testing Tools
- Blockchain Explorers
- Real-World Use Cases of Solidity Programming Language
- Decentralized Finance (DeFi)
- Token Creation
- Decentralized Applications (DApps)
- Common Challenges in Solidity Development
- Debugging Smart Contracts
- Handling Upgradability
- Managing Gas Costs
- Future of Solidity Programming Language
- Upcoming Features
- Alternative Languages for Ethereum
- Conclusion
- Frequently Asked Questions (FAQ)
For more updates, join us on:
WhatsApp TelegramContent:
1. Introduction to Solidity Programming Language
What is Solidity Programming Language?
Solidity is a high-level, statically-typed language designed for creating smart contracts on Ethereum and other blockchain platforms compatible with the Ethereum Virtual Machine (EVM). Created by Gavin Wood in 2014, it is the most widely used language for developing blockchain-based applications.
Why Learn Solidity?
It is the cornerstone of blockchain application development. Mastering it allows developers to create decentralized applications (DApps) and digital tokens, unlocking numerous opportunities in finance, gaming, and more.
2. Key Features of Solidity Programming Language
Object-Oriented Programming
Solidity supports object-oriented programming, which enables modular and reusable code structures.
Smart Contract Language
It is specifically tailored for writing smart contracts that automate processes, enforce agreements, and manage digital assets without intermediaries.
Compatibility with Ethereum Virtual Machine (EVM)
Solidity code compiles into bytecode, which is executed on the Ethereum Virtual Machine (EVM). This ensures that smart contracts run seamlessly on Ethereum-compatible blockchains.
3. Setting Up the Solidity Development Environment
Installing Prerequisites
- Install Node.js and npm for managing dependencies.
- Set up a blockchain node client like Ganache or Hardhat for local testing.
Using Remix IDE
Remix is a web-based integrated development environment (IDE) for Solidity programming. It allows you to write, compile, and deploy smart contracts directly from your browser.
Setting Up Local Development Tools
Use frameworks such as Truffle or Hardhat for a comprehensive development setup. MetaMask is also essential for connecting to blockchain networks.
4. Basics of Solidity Programming Language
Syntax Overview
The Solidity syntax is similar to JavaScript, making it intuitive for developers familiar with C-like languages.
Variables and Data Types
- State Variables: Persistent storage on the blockchain.
- Data Types: uint, int, address, bool, etc.
Example:
uint public count = 0;
address public owner;
Functions and Modifiers
Functions define contract behavior, and modifiers like onlyOwner
help enforce access control.
Example:
function increment() public {
count += 1;
}
modifier onlyOwner() {
require(msg.sender == owner);
_;
}
5. Creating Your First Smart Contract
Step-by-Step Instructions
- Open Remix IDE and create a new file
SimpleStorage.sol
. - Write the following code:
pragma solidity ^0.8.0;
contract SimpleStorage {
uint public data;
function set(uint _data) public {
data = _data;
}
function get() public view returns (uint) {
return data;
}
}
Deploying a Contract on Testnet
- Connect Remix to MetaMask.
- Choose a testnet like Rinkeby.
- Deploy the contract and interact with it.
Interacting with Deployed Contracts
Use the contract address to read or write data through Remix or tools like Web3.js.
6. Advanced Concepts in Solidity Programming Language
Events and Logging
Events log contract activities that can be accessed by external applications.
Example:
event DataChanged(uint oldData, uint newData);
Inheritance in Contracts
Inheritance promotes code reuse by allowing contracts to inherit features from parent contracts.
Example:
contract Parent {
function greet() public pure returns (string memory) {
return "Hello";
}
}
contract Child is Parent {}
Libraries in Solidity
Libraries provide reusable code modules for other contracts.
Example:
library Math {
function add(uint a, uint b) public pure returns (uint) {
return a + b;
}
}
Also Read “What is BlockChain Technology“
7. Best Practices for Solidity Development
Writing Secure Contracts
- Prevent reentrancy attacks.
- Use the checks-effects-interactions pattern.
Gas Optimization Tips
- Reduce storage operations.
- Use constant variables whenever possible.
Code Readability and Documentation
Maintain clear, modular code and provide detailed comments to enhance understanding.
8. Tools for Solidity Developers
Popular Frameworks
- Truffle: Comprehensive development suite for smart contracts.
- Hardhat: Developer-friendly framework for Ethereum.
Testing Tools
- Use Mocha and Chai for testing.
- Simulate local blockchains with Ganache.
Blockchain Explorers
- Etherscan for Ethereum.
- BscScan for Binance Smart Chain.
9. Real-World Use Cases of Solidity Programming Language
Decentralized Finance (DeFi)
Building protocols like Uniswap and Aave for financial applications.
Token Creation
Creating fungible (ERC-20) and non-fungible (ERC-721) tokens.
Decentralized Applications (DApps)
Applications across gaming, supply chains, and voting systems.
10. Common Challenges in Solidity Development
Debugging Smart Contracts
Utilize Remix’s debugger and tools like Tenderly for analyzing transaction failures.
Handling Upgradability
Use proxy patterns to enable updatable contract logic.
Managing Gas Costs
Optimize loops and minimize computational tasks.
11. Future of Solidity Programming Language
Upcoming Features
- Enhanced error handling and type safety.
Alternative Languages for Ethereum
- Vyper: Python-like language focusing on security and simplicity.
12. Conclusion
The Solidity PL is integral to blockchain development. By mastering its tools, syntax, and best practices, developers can create innovative and secure smart contracts for decentralized applications.
13. Frequently Asked Questions (FAQ)
Q1: What is Solidity programming language used for? Solidity is used to develop smart contracts that automate processes and manage digital assets on blockchain networks.
Q2: How can I start learning Solidity? Start with the official Solidity documentation, practice with Remix IDE, and experiment with deploying contracts on testnets.
Q3: Is Solidity P L secure? While Solidity has robust features, developers must follow best practices to ensure contract security and prevent vulnerabilities.
Q4: Can I use Solidity outside Ethereum? Yes, Solidity is compatible with Ethereum-compatible blockchains like Binance Smart Chain and Polygon.
Q5: What tools are essential for Solidity developers? Key tools include Remix IDE, Truffle, Hardhat, MetaMask, and blockchain explorers like Etherscan.
Optimized Keywords:
- Solidity programming language
- Solidity guide
- Smart contract development
- Blockchain programming
- Solidity tools
- Ethereum programming
Blockchain contracts represent a transformative approach to managing agreements. With their ability to automate, secure, and streamline processes, they hold immense potential for reshaping industries. Understanding their intricacies and applications is crucial for businesses and individuals alike.
If you have any questions or inquiries, please feel free to share them in the comments section. Additionally, you can download our official application from the Google Play Store for a seamless experience.